1.普通編程
1.編寫一個程序,調用一次 printf()函數,把你的姓名打印在一行。再調 用一次 printf()函數,把你的姓名分別打印在兩行。而後,再調用兩次printf() 函數,把你的姓名打印在一行。輸出應以下所示(固然要把示例的內容換成 你的姓名):
#include<stdio.h>
int main()
{
printf("Shen zhic\n");
printf("Shen\nzhic\n");
printf("Shen");
printf("zhic");
return 0;
}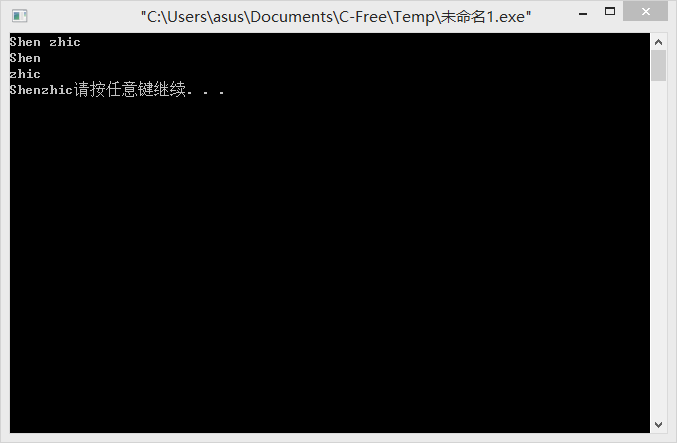
2.編寫一個程序,打印你的姓名和地址。
#include<stdio.h>
int main()
{
printf("shenzhic\n");
printf("浙江桐鄉");
return 0;
}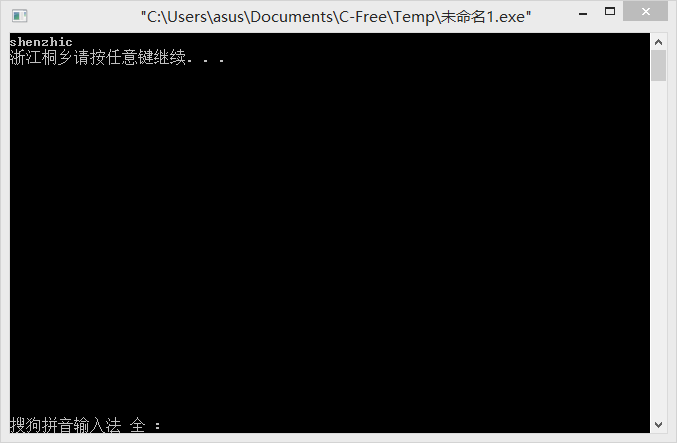
3.編寫一個程序把你的年齡轉換整天數,並顯示這兩個值。這裏不用考 慮閏年的問題。
#include<stdio.h>
int main()
{
int ageyear,ageday,totalageyears;
ageyear=18;
ageday=300;
totalageyears=ageyear*365+ageday;
printf("I am %d years and %d days",ageyear,totalageyears);
return 0;
}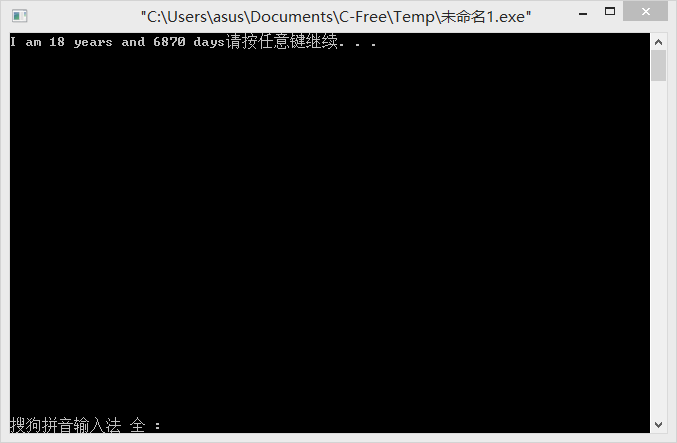
**4.編寫一個程序,生成如下輸出:
For he's a jolly good fellow!
For he's a jolly good fellow!
For he's a jolly good fellow!
Which nobody can deny!
除了 main()函數之外,該程序還要調用兩個自定義函數:一個名爲jolly(),用於打印前 3 條消息,調用一次打印一條;另外一個函數名爲 deny(),打印最後一條消息。******
#include<stdio.h>
void jolly();
void deny();
int main()
{
jolly();
jolly();
jolly();
deny();
return 0;
}
void jolly()
{
printf("For he`s a jolly good fellow!\n");
}
void deny()
{
printf("Which nobody can deny!\n");
}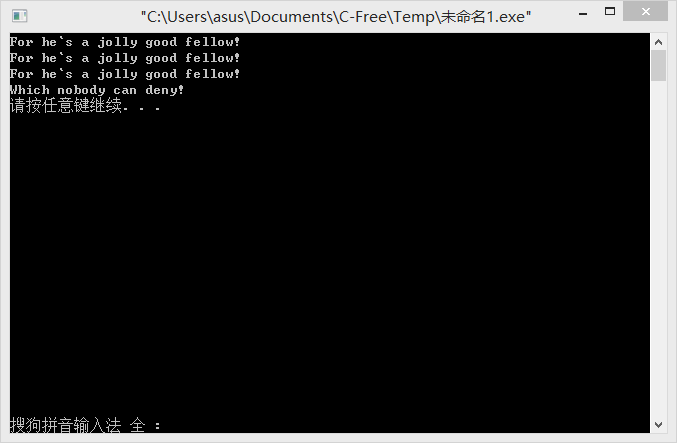
5.編寫一個程序,生成如下輸出:
Brazil, Russia, India, China
India, China,
Brazil, Russia
除了main()之外,該程序還要調用兩個自定義函數:一個名爲br(),調 用一次打印一次「Brazil, Russia」;另外一個名爲ic(),調用一次打印一次「India, China」。其餘內容在main()函數中完成。
#include<stdio.h>
void dh();
void enter();
void br();
void ic();
int main()
{
br();
dh();
ic();
enter();
ic();
enter();
br();
enter();
return 0;
}
void dh()
{
printf(",");
}
void enter()
{
printf("\n");
}
void br()
{
printf("Brazil,Russia");
}
void ic()
{
printf("India,China");
}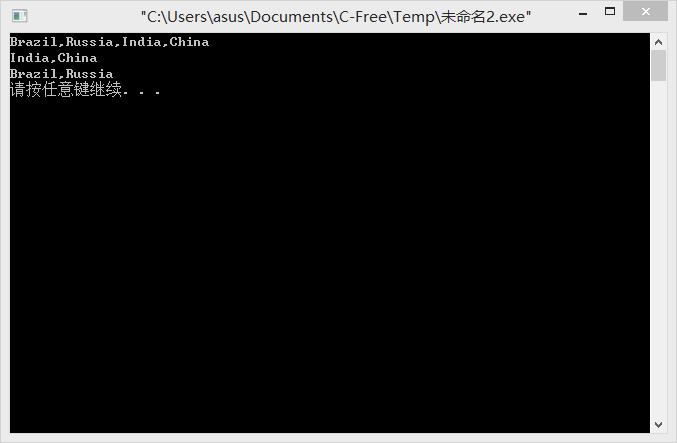
6.編寫一個程序,建立一個整型變量toes,並將toes設置爲10。程序中還 要計算toes的兩倍和toes的平方。該程序應打印3個值,並分別描述以示區 分。
#include<stdio.h>
int main()
{
int toes,dbtoes,pftoes;
toes=10,dbtoes=toes*2,pftoes=toes*toes;
printf("%dtoes\n%ddbtoes\n%dpftoes\n",toes,dbtoes,pftoes);
return 0;
}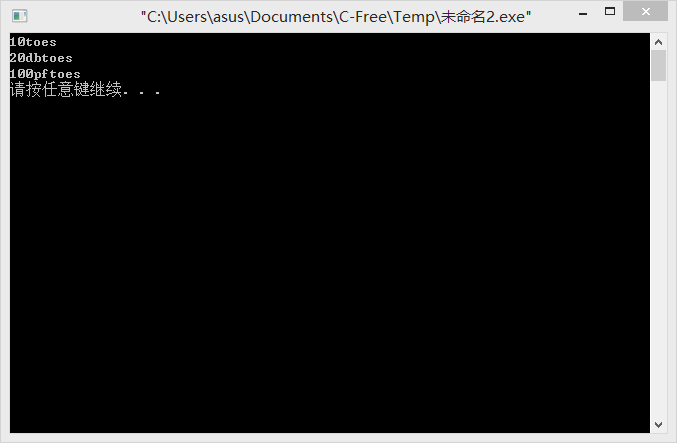
7.許多研究代表,微笑益處多多。編寫一個程序,生成如下格式的輸
出:
Smile!Smile!Smile!
Smile!Smile!
Smile!
該程序要定義一個函數,該函數被調用一次打印一次「Smile!」,根據程 序的須要使用該函數。
#include<stdio.h>
void s();
void enter();
int main()
{
s(),s(),s();
enter();
s(),s();
enter();
s();
enter();
return 0;
}
void s()
{
printf("Smile!");
}
void enter()
{
printf("\n");
}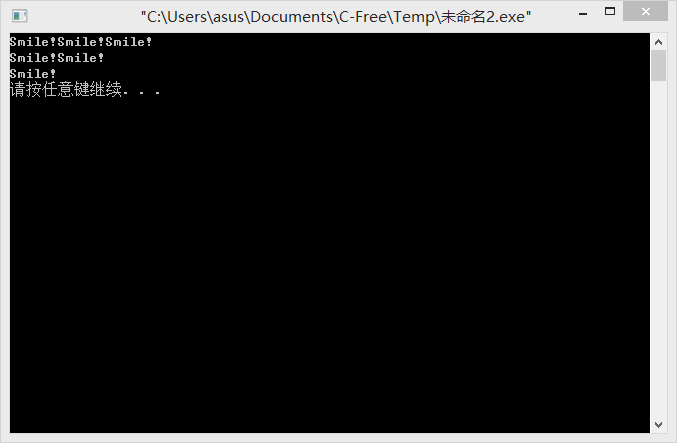
8.在C語言中,函數能夠調用另外一個函數。編寫一個程序,調用一個名 爲one_three()的函數。該函數在一行打印單詞「one」,再調用第2個函數 two(),而後在另外一行打印單詞「three」。two()函數在一行顯示單詞「two」。 main()函數在調用 one_three()函數前要打印短語「starting now:」,並在調用完
107
畢後顯示短語「done!」。所以,該程序的輸出應以下所示:
starting now:
one
two
three
done!
#include<stdio.h>
void one_three();
void two();
int main()
{
printf("starting now:\n");
one_three();
printf("done!\n");
return 0;
}
void one_three()
{
printf("one\n");
two();
printf("three\n");
}
void two()
{
printf("two\n");
}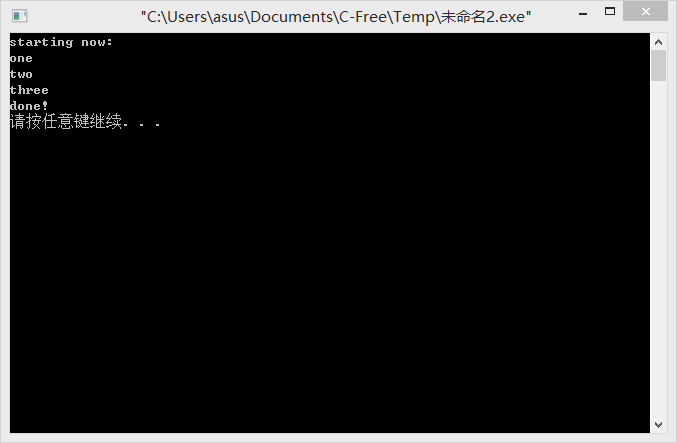