題目
Given a string containing just the characters '(',')','[',']','{' and ‘}’,determine if the input string is valid.
An input string is valid if:python
- 1.Open brackets must be closed by the same type of brackets.
- 2.Open brackets must be closed in the correct order.
Note that an empty string is also considered valid.
Example 1:
Input:"()"
Output:true
Example 2:
Input:"()[]{}"
Output:true
Example 3:
Input:"(]"
Output:false
Example 4:
Input:"([)]"
Output:false
Example 5:
Input:"{[]}"
Output:trueide
思路
leetcode上的解答是這樣的:
先考慮簡單的狀況:只有一種括號.net
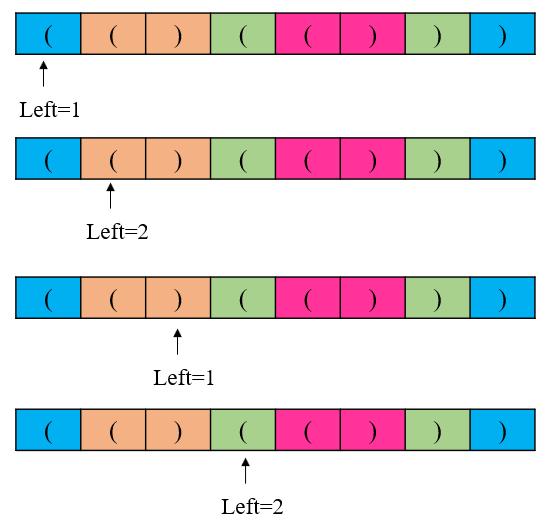
圖1:只有一種括號
定義一個計數器left,若是咱們遇到一個開括號,則計數器left加1;若是遇到一個閉括號,此時有兩種狀況,一是當前left=0,這說明沒有配對的開括號,表達式無效;二是left>0,說明還有沒配對的開括號,left--。 可是實際狀況不止一種括號,可是咱們發現,爲了保證表達式的每個子表達式都是也是有效的,每個閉括號老是與左起最近的開括號是匹配的,如圖2。

圖2:多個括號的狀況
這個時候就要用到棧。在表示問題的遞歸結構時,因爲咱們不清楚總體的結構,沒法從裏到外的解決問題,此時棧能夠幫助咱們遞歸地解決問題,從外到內地解決問題。 因而本題的解題思路以下: * 1.初始化一個堆棧,並初始化一個映射表(用來處理不一樣種類的括號); * 2.循環處理全部括號 * 若是是開括號,則push進棧便可; * 若是是閉括號,那麼就須要檢查棧頂的元素,若是棧頂的開括號與當前的閉括號匹配(經過查找創建的映射表匹配),則pop出棧;若是不匹配,說明表達式無效;
- 3.當處理完全部括號後,若是棧中還有元素,說明表達式無效。
就想在玩一個消消樂,只有當開括號和閉括號匹配的時候才能消掉這一對括號。
Tips
棧
一種線性存儲表。它有如下兩種特性:3d
- 棧中數據按照後進先出(last-in, first-out, LIFO)的方式進棧和出棧的。
- 只能從棧頂(top)操做數據。
咱們能夠把它想象成幾個盤子堆在彈簧上的場景。code
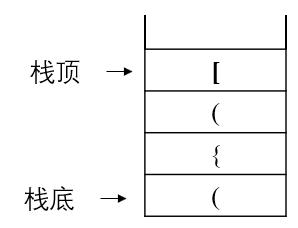
圖4:棧的結構示意圖
**入棧(push)**
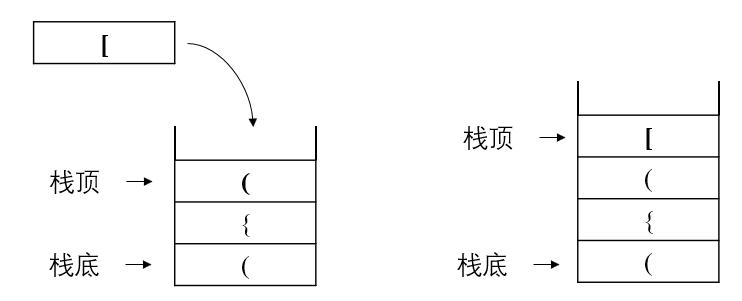
圖5:入棧示意圖
**出棧(pop)**
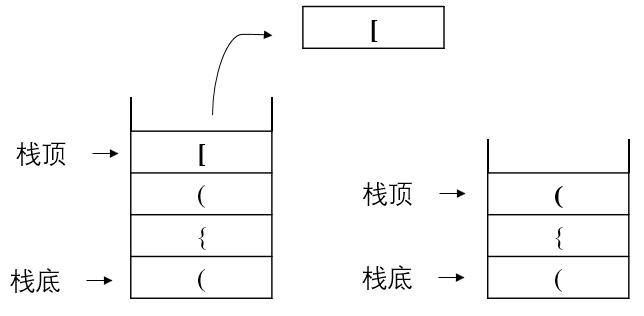
圖6:出棧示意圖
C++
bool isValid(string s) {
map<char,char> table={ {')', '('}, {']', '['}, {'}', '{'} };
if( s.size()== 0 )
return true;
stack<char> cStack;
for(int i = 0;i<s.size(); i++){
if(s[i] == '(' || s[i] == '[' || s[i] == '{')
cStack.push(s[i]);
else if(s[i] == ')' || s[i] == ']' || s[i] == '}'){
if(cStack.empty())
return false;
char popChar = cStack.top();
if(popChar == table[s[i]]){
cStack.pop();
continue;
}
else
return false;
}
}
if(cStack.empty())
return true;
return false;
}
Python