照例附上項目github連接java
本項目實現的是將一個簡單的天氣預報系統一步一步改形成一個SpringCloud微服務系統的過程。本章主要講解微服務的消費。git
微服務的消費模式主要有:github
下面咱們主要講解客戶端發現模式,以及服務端發現模式。web
客戶端發現模式的具體流程以下:算法
1)服務實例啓動後,將本身的位置信息提交到服務註冊表中。spring
2)客戶端從服務註冊表進行查詢,來獲取可用的服務實例。編程
3)客戶端自行使用負載均衡算法從多個服務實例中選擇出一個。app
服務端發現模式與客戶端發現模式的區別在於:服務端發現模式的負載均衡由負載均衡器(獨立的)來實現,客戶端不須要關心具體調用的是哪個服務。負載均衡
下面咱們價格介紹一些常見的微服務的消費者。分佈式
HttpClient是常見的微服務的消費者,它是Apache Jakarta Common 下的子項目,能夠用來提供高效的、最新的、功能豐富的支持 HTTP 協議的客戶端編程工具包,而且它支持 HTTP協議最新的版本和建議。
Ribbon是一個基於HTTP和TCP的客戶端負載均衡工具,它基於Netflix Ribbon實現。經過Spring Cloud的封裝,可讓咱們輕鬆地將面向服務的REST模版請求自動轉換成客戶端負載均衡的服務調用。
Ribbon常與Eureka結合使用。在典型的分佈式部署中,Eureka爲全部的微服務提供服務註冊,Ribbon提供服務消費的客戶端,含有許多負載均衡的算法。
Feign是一個聲明式的Web Service客戶端,它的目的就是讓Web Service調用更加簡單。Feign提供了HTTP請求的模板,經過編寫簡單的接口和插入註解,就能夠定義好HTTP請求的參數、格式、地址等信息。
而Feign則會徹底代理HTTP請求,咱們只須要像調用方法同樣調用它就能夠完成服務請求及相關處理。Feign整合了Ribbon和Hystrix(關於Hystrix咱們後面再講),可讓咱們再也不須要顯式地使用這兩個組件。
總起來講,Feign具備以下特性:
本節咱們主要講解如何集成Feign,實現微服務的消費功能。
pom.xml配置文件,添加以下依賴。
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-feign</artifactId> </dependency>
application.yml文件,添加以下配置
服務的名稱與工程的名稱一致,而且設置請求一個服務的connect與read的超時時間。
spring: application: name: micro-weather-eureka-client-feign eureka: client: service-url: defaultZone: http://localhost:8761/eureka/ feign: client: config: feignName: connectTimeout: 5000 readTimeout: 5000
添加@EnableFeignClients註解,啓動Feign功能。
package com.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.web.servlet.ServletComponentScan; import org.springframework.cloud.client.discovery.EnableDiscoveryClient; import org.springframework.cloud.netflix.feign.EnableFeignClients; import org.springframework.scheduling.annotation.EnableScheduling; //@ServletComponentScan(basePackages="com.demo.web.servlet") @SpringBootApplication @EnableDiscoveryClient @EnableScheduling @EnableFeignClients public class Sifoudemo02Application { public static void main(String[] args) { SpringApplication.run(Sifoudemo02Application.class, args); } }
建立一個Feign客戶端,經過其CityClient的接口,能夠調用城市數據API微服務mas-weather-city-eureka中的cities接口,返回城市列表。
package com.demo.service; import java.util.List; import org.springframework.cloud.netflix.feign.FeignClient; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import com.demo.vo.City; //建立一個Feign客戶端 獲取城市數據微服務中的城市列表信息 @FeignClient("msa-weather-city-eureka") public interface CityClient { @RequestMapping(value="/cities",method=RequestMethod.GET) List<City> listCity()throws Exception; }
@RestController public class CityController{ @Autowired private CityClient cityClient; @GetMapping("/cities") public List<City> listCity(){ List<City> cityList=cityClient.listCity(); 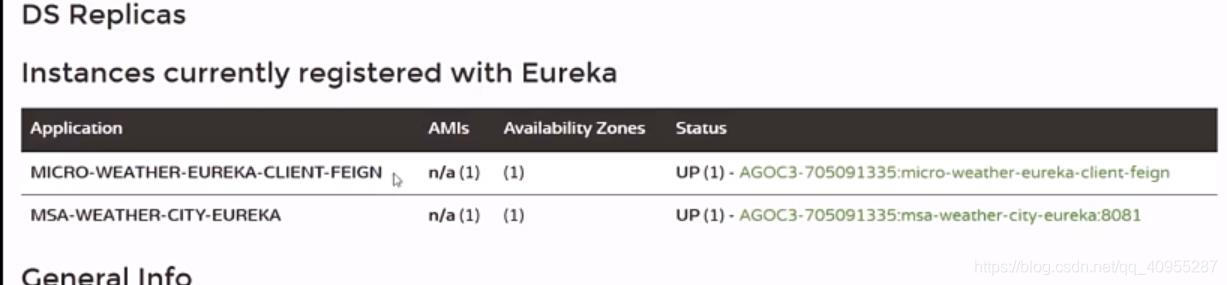 return cityList; } }
先啓動Eureka服務端,而後啓動被請求的微服務——城市數據API微服務,最後啓動Feign客戶端。